Signals¶
At some point you will want to tell a bot to stop processing jobs. There are a number of ways to do this which give you control over when and how the bot and the workers will behave.
terminate
¶
Warm termination¶
Use this if you want a worker to finish the job that it is doing and then exit.
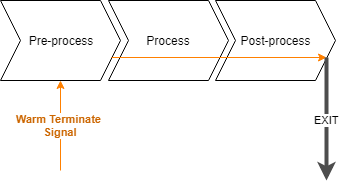
Cold termination¶
Use this if you want a worker to exit as soon as the current step function is complete.
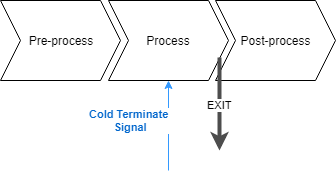
stop
¶
Stopping a worker will allow the worker to finish the current job but will not ask for any more jobs. However, unlike a terminate call, the worker will not exit and will be available to resume later.
resume
¶
When a worker is stopped, calling resume will cause it to start processing jobs again.
Sending signals¶
Workers can be sent signals through the bot or worker on a session:
import time
from pyqalx import QalxSession
qalx = QalxSession()
my_bot = qalx.bot.find_one(name="My Bot")
qalx.bot.terminate(my_bot) # does a warm termination on all workers on the bot
bad_bot = qalx.bot.find_one(name="My Naughty Bot")
qalx.bot.terminate(bad_bot, warm=False) # does a cold termination of all workers on the bot
busy_bot = qalx.bot.find_one(name="My Busy Bot")
first_worker = busy_bot.workers[0]
qalx.worker.terminate(first_worker) # does a warm termination on only a single worker
second_worker = busy_bot.workers[1]
qalx.worker.stop(second_worker) # tells another worker to stop processing
time.sleep(600) # have a rest for 10 mins, coffee anyone?
qalx.worker.resume(second_worker) # crack on